Dart小记
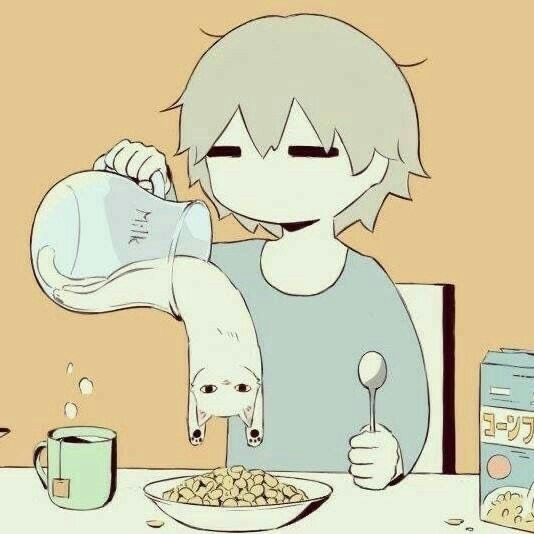
泛型方法:
- dart 的泛型方法书写和 Java 的不太一样。
- Dart 的泛型方法
1 | T first<T>(List<T> ts) { |
Tips
- Java 的泛型方法
1 | <T> T first(List<T> ts) { |
Mixin(混入)
Tips
作用:解决无需多重继承即可拥有功能方法
混合使用 with 关键字,with 后面可以是 class、abstract class 和 mixin 的类型
1 | // mixin 声明的类 |
- 混入(with)多类,遇到同名方法的情况,按照混入的顺序,后面的会覆盖前面
- mixin 的类无法定义构造函数,所以一般会将需要 mixin 的类使用 mixin 关键字
- 使用关键字 on 限定混入条件
1 | class Person { |
- 混合后的类型是超类的子类型(类似多继承)
1 | class F { |
类
- 调用非默认超类构造函数
1 | class Person { |
1 | class Vector2d { |
- Title: Dart小记
- Author: Jared Yuan
- Created at : 2023-09-22 11:41:25
- Updated at : 2023-09-22 12:07:32
- Link: https://redefine.ohevan.com/2023/09/22/Dart小记/
- License: This work is licensed under CC BY-NC-SA 4.0.